19+ High-Frequency Trading (HFT) Strategies
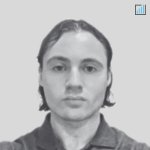
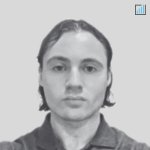
High-frequency trading (HFT) involves the use of sophisticated algorithms and high-speed data networks to execute orders at extremely fast speeds.
HFT strategies are designed to capitalize on very small price discrepancies that exist for a very short time (often a fraction of a second).
Given the technical nature of HFT, the strategies often require an understanding of market microstructure, advanced mathematical models, and extensive technological infrastructure.
Here are some common HFT models and strategies:
Market Making
This strategy involves continuously buying and selling securities to provide liquidity to the market.
HFT market makers aim to profit from the spread between the bid and ask prices, responding quickly to changes in supply and demand.
Arbitrage
This involves exploiting price discrepancies across different markets or different securities.
Examples include:
- Index Arbitrage – Exploiting price differences between a stock index and a futures contract on that index.
- Statistical Arbitrage – Using statistical models to identify price discrepancies between similar or related securities.
- Triangular Arbitrage – Exploiting price differences between three currencies in the foreign exchange market.
Latency Arbitrage
This strategy takes advantage of delays in the dissemination of market data.
Traders with the fastest connections can receive and act on data before other market participants.
Latency is also the primary reason why most HFT algorithms are traditionally written in C++.
C++ is a compiled language that takes less time for a computer to interpret it, which results in traditionally faster speeds than higher-level languages like Python.
Event Arbitrage
This strategy involves trading securities based on anticipated events such as earnings reports, regulatory changes, or M&A announcements.
Algorithms predict the market’s reaction to these events and execute trades at high speeds.
Order Flow Prediction
Some HFT strategies try to predict the future orders of large institutional trades.
By detecting patterns or signals that precede large trades, HFT algorithms can position themselves advantageously.
Momentum/Ignition Strategies
These strategies involve identifying and following early signs of market movement in a particular direction and then trading aggressively in that direction, often leading to a “momentum ignition” where the movement becomes self-sustaining for a short period.
Quote Stuffing
This controversial strategy involves placing and then quickly canceling large numbers of orders.
This creates “noise” or confusion in the market to gain an advantage.
Flash Orders
This involves placing orders (typically only available for a fraction of a second) that are visible to a select group of traders before they are available to the entire market.
Spoofing and Layering
These are illegal strategies where traders place orders with no intention of executing them to create a misleading impression of market sentiment.
Volume-Weighted Average Price (VWAP) Tracking
This strategy involves executing orders in a way that aims to match or beat the VWAP of a stock over a specific time frame.
HFT firms use this strategy to provide VWAP matching services to large institutional traders.
It’s also commonly used more generally when a big or complicated trade needs to be made.
Essentially, it helps get out of a position while minimizing market disruption and transaction costs.
Regulatory Arbitrage
This involves taking advantage of differences in regulations across regions or markets.
Algorithms are designed to spot and exploit these gaps.
Time-Weighted Average Price (TWAP) Strategy
Similar to VWAP, but the focus is on distributing trades evenly across a specified time period (rather than volume) to minimize market impact.
Order Book Imbalance
This strategy involves analyzing the real-time supply and demand in the market by closely monitoring the order book (aka Level II data).
The goal is to identify short-term price movements based on the imbalance of buy and sell orders.
Mean Reversion Strategies
This involves algorithms that identify and exploit small deviations from a security’s historical price trends.
The assumption is that prices will revert to their mean or average level after these small deviations.
Tick Data Strategies
Using the granular level of tick data (every change in price, no matter how small), these strategies can be used to detect patterns or trends that are invisible in higher time frame data.
Microstructure Noise Exploitation
Some HFT algorithms are designed to exploit “noise” in the market – small, seemingly random fluctuations in prices – which are often ignored by traditional trading strategies.
Co-Location and Proximity Hosting
While not a trading strategy per se, the practice of placing servers physically close to the exchange’s servers (co-location) or using proximity hosting services to reduce data transmission time is a key enabler for many HFT strategies.
Signal-Based Strategies
These involve algorithms that act on signals from a variety of data sources.
This includes news feeds, social media, economic reports, etc., at high speed to trade ahead of anticipated price moves.
Machine Learning and AI-Based Strategies
Some HFT firms use machine learning algorithms and artificial intelligence to predict market movements, identify trading opportunities, or optimize existing trading strategies.
Dark Pool Liquidity Detection
Some HFT strategies focus on detecting the presence of large hidden orders in dark pools and trading ahead of these orders in public markets.
What Does a HFT Algorithm Look Like?
Most HFT algorithms and systems are done in C++.
C++ is a lower-level language that’s compiled and there’s less need to interpret compared to a higher-level language like Python.
(Related: C++ vs. Python for Finance)
This example will focus on a simple statistical arbitrage strategy between SPY (an ETF that tracks the S&P 500) and the underlying stocks of the S&P 500.
The core idea is to identify temporary mispricings between SPY and a subset of its constituent stocks, and exploit these for profit.
Creating an HFT algorithm in C++ for statistical arbitrage involves a complex process.
While we can’t provide a complete, production-ready code due to the complexity and the customization required for each trading strategy and environment, we can outline a basic conceptual example.
We’ll walk through it with comments along the way.
#include <iostream> #include <vector> #include <map> #include <string> // This is simplified. Real-world implementation would require // a more sophisticated setup, including a real-time market data feed, // execution system, risk management, etc. class Stock { public: std::string ticker; double price; double weight; // Weight of the stock in the SPY ETF // ... Other relevant data }; class MarketDataFeed { // This would handle real-time data updates for SPY and individual stocks // In practice, this would connect to a data provider API public: void updateStockPrice(std::string ticker, double price); void updateSPYPrice(double price); // ... Other methods as needed }; class StatisticalArbitrageStrategy { std::map<std::string, Stock> stocks; double spyPrice; MarketDataFeed dataFeed; double calculateSyntheticSPY() { double syntheticPrice = 0.0; for (const auto& pair : stocks) { syntheticPrice += pair.second.price * pair.second.weight; } return syntheticPrice; } void onMarketDataUpdate() { double syntheticSPY = calculateSyntheticSPY(); if (std::abs(spyPrice - syntheticSPY) > some_threshold) { executeArbitrage(spyPrice, syntheticSPY); } } void executeArbitrage(double realSPY, double syntheticSPY) { if (syntheticSPY > realSPY) { // Execute trade: Buy SPY, Short selected stocks } else { // Execute trade: Sell SPY, Long selected stocks } } // ... Other methods and logic }; int main() { // Initialize MarketDataFeed, load initial data for stocks, etc. // Start the strategy }
Important Points
Conclusion
High-frequency trading (HFT) encompasses a range of strategies, many of which are highly technical and specialized.
Beyond the more commonly known strategies like stat arb and market making, several advanced and less well-known HFT strategies focus on exploiting very specific market dynamics or technological edges.
These strategies typically require sophisticated algorithms, specialized knowledge, and a deep understanding of market microstructure.
Furthermore, the success of these strategies often depends on the ability to process and analyze large volumes of data at extremely high speeds.
Due to the complexity and resources required, these strategies are typically the domain of well-funded institutional traders or specialized HFT firms, as it requires significant investment in technology, data, and expertise.