How to Setup a Trading Algorithm in C++
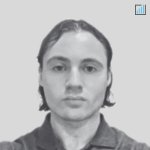
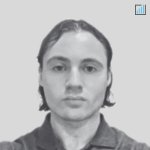
Setting up a trading algorithm in C++ and connecting it to a broker for live trading involves several steps and considerations.
In the first part of the article, we’ll include a high-level overview of the process.
In the second part, we’ll go into more specifics on where to write the algorithm/trading system and how to connect it to your broker.
Both parts will slightly overlap.
1. Developing the Trading Algorithm
Define Strategy
Begin by defining your trading strategy.
This could be based on technical indicators, statistical analysis, fundamental data, machine learning models, or a combination of these.
It could even be as simple as asking the algorithms to keep a portfolio within the allocation
Coding the Algorithm
Implement your strategy in C++.
This will involve writing functions to process market data, generate trading signals, and manage orders.
Backtesting
Rigorously test your algorithm with historical data to ensure it performs as expected.
Forward Testing
Also strongly consider forward testing (i.e., stress testing) it to see how it holds up in extreme scenarios.
This phase is important for identifying and fixing bugs, and for validating the strategy’s effectiveness.
2. Selecting a Broker
Broker Compatibility
Ensure that your chosen broker supports algorithmic trading and offers a way to connect custom trading algorithms, typically through an API (Application Programming Interface).
API Documentation
Obtain and study the broker’s API documentation.
This is used for understanding how to programmatically interact with the broker’s trading platform.
Independent API Services
There are also independent services you can use to connect your algorithms to your broker directly.
Nonetheless, many don’t support C++.
QuantConnect, for example, only supports Python and C#.
You can always program in a separate language, but Python is known for being slower as a high-level language (i.e., requires more interpretation and is less suited for trading systems where speed is an important consideration).
3. Connecting to the Broker’s API
API Integration
Use the broker’s API to connect your trading algorithm to the broker’s trading platform.
This will involve handling authentication, streaming market data, sending orders, and managing account information.
Language Compatibility
Ensure the broker’s API is compatible with C++ or provides a way to interact with C++ applications, such as through RESTful APIs, WebSockets, or other methods.
Direct Market Access (DMA)
Some platforms and brokers offer DMA.
This gives your algorithm direct access to the exchange order book for faster execution.
This option typically requires higher trading volume and experience.
4. Setting Up a Trading Environment
Safe Testing Environment
Initially, set up your algorithm to trade in a simulated or paper trading environment provided by the broker.
This allows you to test the integration without financial risk.
Server and Connectivity
Consider running your algorithm on a dedicated server with a reliable and fast internet connection – especially if you require low latency.
5. Risk Management
Implement Risk Controls
Incorporate strict risk management rules into your algorithm.
Monitoring
Even with automated trading, regular monitoring is important to ensure the system operates as intended and to intervene in case of unexpected market events or technical issues.
6. Going Live
When you switch to live trading, start with a small amount of capital to test the algorithm’s performance in real market conditions.
Continuously monitor the algorithm’s performance and make adjustments as needed based on live trading data and changing market conditions.
Other Considerations
Compliance and Legal
Ensure your trading activities comply with any regulatory requirements.
Depends (mostly) on your location and assets traded.
Security
Pay attention to security aspects, especially related to API keys and personal data.
If you use a third-party service, is your data encrypted?
Costs
Be aware of transaction costs and how they might affect your strategy’s profitability.
API Rate Limits
Be aware of and respect the API rate limits set by the broker.
Error Handling
Implement robust error handling to deal with network issues, API errors, or unexpected market conditions.
Technical Skills
C++ Proficiency
You should have a good grasp of C++ programming, including:
- handling real-time data
- multi-threading, and
- network programming
Financial Knowledge
Understanding of financial markets and instruments is important for developing and refining trading strategies.
Now let’s get into more specifics on writing the algorithm or trading system and how to connect it to your broker.
(Some of this will overlap with what we said above.)
Writing a trading algorithm and connecting it to a broker involves both development and integration steps.
Writing the Trading Algorithm or System
Development Environment Setup
- Choose an IDE – Use an Integrated Development Environment (IDE) like Visual Studio, Eclipse, or Code::Blocks that supports C++ development.
- Install Necessary Libraries – Depending on your trading strategy, you might need libraries for statistical analysis, machine learning, or data processing (e.g., Boost, Eigen, TensorFlow for C++).
- Version Control – Use version control systems like Git for tracking changes and managing different versions of your algorithm.
Algorithm Development
- Strategy Formulation – Define your trading strategy.
- Coding – Write the algorithm in C++. This involves creating functions for market data analysis, signal generation, risk management, and order execution.
- Backtesting – Use historical data to test your algorithm. This step is important to validate the strategy before going live.
Connecting to Broker
Broker API Selection
- Choose a Broker – Select a broker that offers API access for automated trading. Popular choices for algorithmic trading include Interactive Brokers, TD Ameritrade, and Alpaca.
- API Documentation – Obtain and thoroughly understand the broker’s API documentation. This will provide details on how to establish a connection, stream market data, execute trades, and manage your account.
API Integration
- Library/SDK Installation – Install any client libraries or SDKs provided by the broker for C++ or a compatible language.
- Authentication – Implement the authentication mechanism to securely connect to the broker’s API.
- Function Integration – Develop functions to handle API requests for retrieving market data, sending orders, and managing the portfolio.
Testing Integration
- Paper Trading – Most brokers offer a sandbox or paper trading environment. Test your algorithm in this simulated environment to validate the integration without risking real capital.
- Handling Real-time Data – Ensure your system can handle real-time data feed and execute orders with the required latency.
Deployment
Trading Environment
- Server Setup – Consider deploying your algorithm on a reliable server, possibly near your broker’s server for low latency.
- Secured Connection – Ensure your connection to the broker’s API is secure (especially if trading over the internet).
Monitoring and Maintenance
- Live Monitoring – Regularly monitor the algorithm’s performance and system health.
- Updates and Adjustments – Be prepared to update your algorithm in response to issues encountered during live trading or any improvements you wish to make.
Conclusion
Developing and deploying a live trading algorithm is a complex and nuanced process that requires a blend of programming expertise, financial knowledge, and rigorous testing.
It’s important to approach it methodically and cautiously, given the potential financial risks involved.
Related