Python vs. C++ for Financial Algorithms
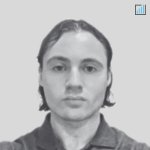
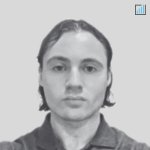
When comparing Python and C++ for financial algorithms, particularly in quantitative finance and economics, it’s important to consider several factors, including:
- performance
- ease of use
- library support, and
- the specific requirements of the task at hand
We’ll go through each category by category.
Key Takeaways – Python vs. C++ for Financial Algorithms
- Performance:
- C++ excels in high-performance scenarios like high-frequency trading.
- Python is more suitable for rapid development and less performance-critical tasks.
- Ease of Use:
- Python offers greater simplicity and a rich library ecosystem – ideal for data analysis and prototyping.
- C++ requires deeper technical expertise.
- Application:
- Python is preferred for data analysis and machine learning due to extensive libraries.
- C++ is chosen for optimizing performance-critical parts of financial models.
Performance
C++
Known for its high performance due to its ability to execute close to the hardware.
This is important in high-frequency trading where latency can be a decisive factor.
C++ allows for more control over memory management and system resources, which can lead to faster execution times for complex calculations.
Python
Generally slower than C++ due to being an interpreted language – i.e., translated into machine code at the time of execution (rather than before) and read line by line.
But Python’s performance can be significantly enhanced by using libraries like NumPy and Pandas, which are written in C and optimized for speed.
Ease of Use and Development Speed
Python
Known for its readability and simplicity.
This makes it an excellent choice for rapid development and prototyping.
Python’s syntax is clear and concise, which allows for faster coding and debugging.
This is an advantage in dynamic environments where financial models often need to be updated or modified.
C++
More complex and verbose compared to Python.
It requires a deeper understanding of memory management and system architecture.
This can increase the development time.
Library Support and Ecosystem
Python
Has a rich ecosystem of libraries specifically designed for data analysis, machine learning, and quantitative finance (e.g., NumPy, Pandas, SciPy, scikit-learn, TensorFlow, Keras).
This extensive library support can significantly reduce the time needed to implement complex financial models.
C++
Has libraries for quantitative finance (like QuantLib).
But the ecosystem is not as extensive as Python’s.
Integration with machine learning libraries is also less straightforward.
Specific Use Cases
High-Frequency Trading
C++ is often preferred due to its higher performance and lower latency, which are critical in this domain.
Data Analysis and Machine Learning
Python is generally preferred due to its simplicity, readability, and the vast array of libraries available for data analysis and machine learning.
Complex Financial Modeling
Both languages are used, but Python is often chosen for development and prototyping, while C++ is used to optimize parts of the code that require high performance.
Integration and Flexibility
Python
Can easily be integrated with other languages and technologies.
It’s often used as a “glue” language in complex systems.
C++
Offers more control and can be used in performance-critical parts of a system.
But it’s less flexible in terms of integration compared to Python.
Python Syntax vs. C++ Syntax
Let’s look at a Monte Carlo simulation where we simulate a portfolio’s return after one year.
We’ll do it first in Python, then in C++.
Python
import numpy as np import matplotlib.pyplot as plt # Monte Carlo simulation for forward testing a specific portfolio's distribution over one year # Portfolio composition with allocations, expected returns, and volatilities portfolio = { "Stocks": {"allocation": 0.35, "return": 0.06, "volatility": 0.15}, "Bonds": {"allocation": 0.40, "return": 0.04, "volatility": 0.10}, "Cash": {"allocation": 0.10, "return": 0.03, "volatility": 0}, "Gold": {"allocation": 0.15, "return": 0.03, "volatility": 0.15} } # Simulation parameters num_simulations = 10000 # Number of Monte Carlo simulations time_horizon = 1 # Time horizon for the simulation (1 year) initial_investment = 100000 # Initial investment amount # Simulating portfolio value over the time horizon portfolio_values = np.zeros(num_simulations) np.random.seed(0) # For reproducibility for i in range(num_simulations): final_value = initial_investment # Calculating portfolio value at the end of time horizon for each asset for asset, info in portfolio.items(): annual_return = info["return"] annual_volatility = info["volatility"] random_return = np.random.normal(annual_return, annual_volatility) final_value += final_value * info["allocation"] * random_return portfolio_values[i] = final_value # Plotting the distribution of portfolio values with adjusted scale plt.hist(portfolio_values, bins=50, color='blue', edgecolor='black') plt.title('Simulated Portfolio Value Distribution after 1 Year') plt.xlabel('Portfolio Value') plt.ylabel('Frequency') plt.show()
In your IDE, the code, with proper indentations, might appear as:
The histogram would appear as such:
C++
In C++, we might set this up as:
#include <iostream> #include <vector> #include <random> #include <algorithm> #include <numeric> #include <map> #include <string> // Structure to represent each asset in the portfolio struct Asset { double allocation; double expected_return; double volatility; }; int main() { // Portfolio composition with allocations, expected returns, and volatilities std::map<std::string, Asset> portfolio = { {"Stocks", {0.35, 0.06, 0.15}}, {"Bonds", {0.40, 0.04, 0.10}}, {"Cash", {0.10, 0.03, 0.00}}, {"Gold", {0.15, 0.03, 0.15}} }; // Simulation parameters const int num_simulations = 10000; const int time_horizon = 1; const double initial_investment = 100000; // Setting up random number generation std::mt19937 generator(10); // For reproducibility std::normal_distribution<double> distribution(0.0, 1.0); // Simulating portfolio values std::vector<double> portfolio_values(num_simulations, 0.0); for (int i = 0; i < num_simulations; ++i) { double final_value = initial_investment; for (const auto& [key, asset] : portfolio) { double random_return = asset.expected_return + asset.volatility * distribution(generator); final_value += final_value * asset.allocation * random_return; } portfolio_values[i] = final_value; } // Calculating Value at Risk (VaR) and Conditional Value at Risk (CVaR) std::sort(portfolio_values.begin(), portfolio_values.end()); double VaR_95 = portfolio_values[num_simulations * 5 / 100]; double CVaR_95 = std::accumulate(portfolio_values.begin(), portfolio_values.begin() + num_simulations * 5 / 100, 0.0) / (num_simulations * 5 / 100); // Outputting VaR and CVaR std::cout << "Value at Risk (VaR) at 95% confidence level: $" << VaR_95 << std::endl; std::cout << "Conditional Value at Risk (CVaR) at 95% confidence level: $" << CVaR_95 << std::endl; return 0; }
It takes slightly longer to do this in C++ than Python (about 20% more code).
However, each programmer might do this a bit differently.
How do the results compare?
Given that these are Monte Carlo simulations, they should be very similar but slightly different.
From our Python code we get:
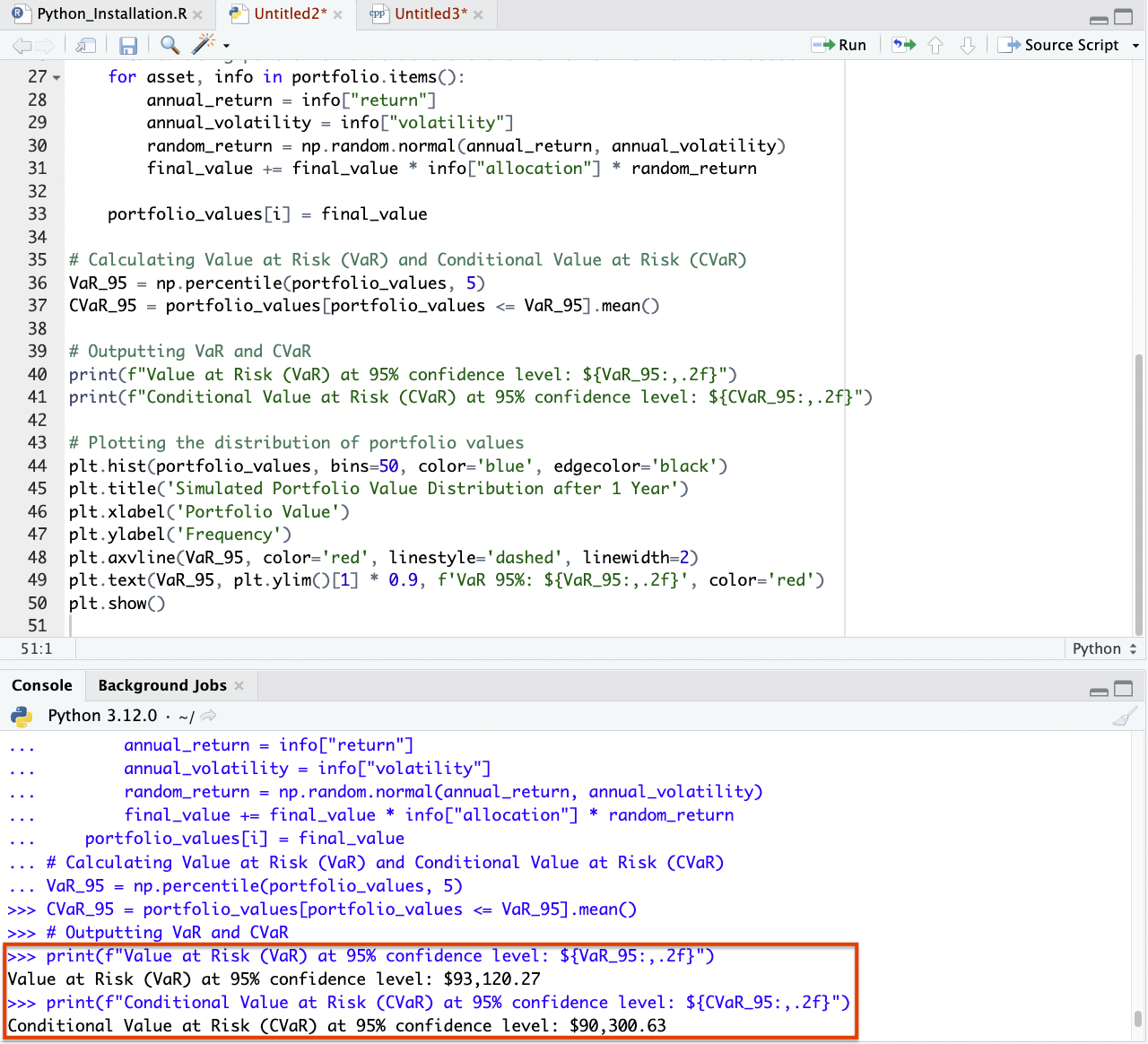
From our C++ model we get:
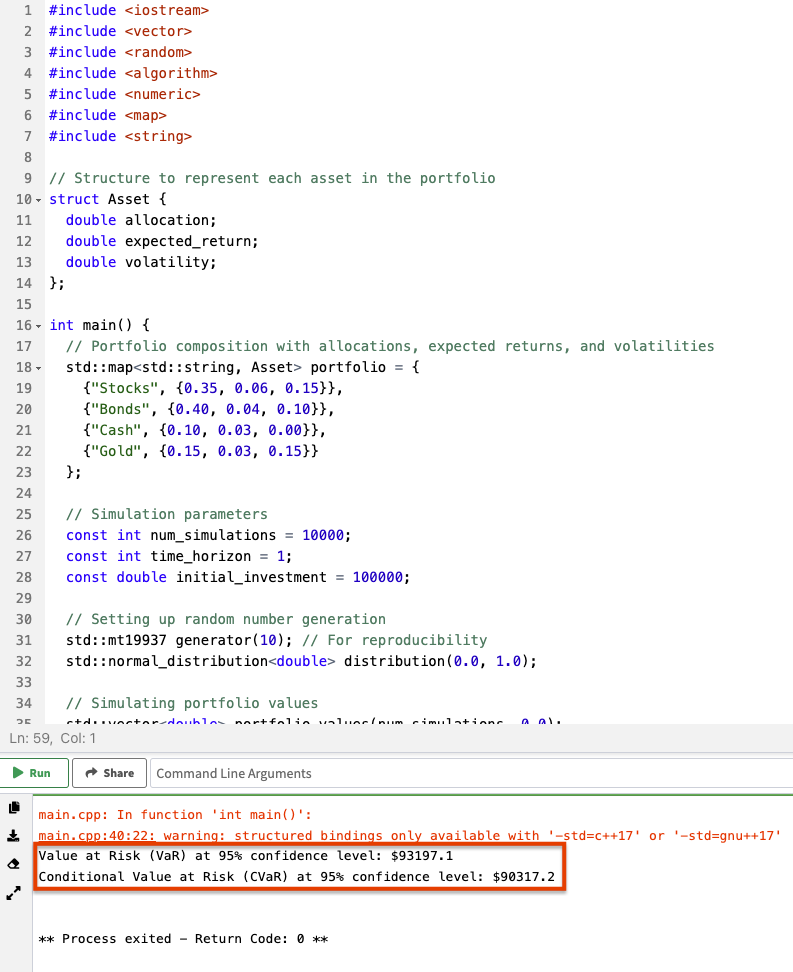
We can see from each that they’re very, very close.
FAQs – Python vs. C++ for Financial Algorithm Development
What are the key differences between Python and C++ in terms of performance for financial algorithms?
Python and C++ differ significantly in performance characteristics, which is especially relevant in financial algorithms.
C++ is a compiled language, meaning it converts code directly into machine language, which is executed by the computer’s hardware.
This results in faster execution times, making C++ highly efficient for compute-intensive tasks such as high-frequency trading algorithms, where microseconds matter.
Python, being an interpreted language, generally exhibits slower execution times because the Python interpreter translates code into machine language at runtime.
Nonetheless, Python’s performance can be enhanced by:
- using libraries like NumPy, which perform heavy computations in C, and
- tools like Cython, which allow Python code to be compiled into C for performance-critical sections
Despite this, Python’s inherent performance is still lower compared to the raw speed of C++.
How does the ease of use and learning curve compare between Python and C++ for financial modeling?
Python is widely regarded as more user-friendly and easier to learn than C++.
It’s often called a “batteries included” language – meaning it comes with a lot of features in its code that you don’t have to implement yourself (e.g., memory management).
Its syntax is straightforward, and it’s designed to be highly readable, which is important for rapid development and maintenance of financial models.
Python’s simplicity allows for quicker development cycles, easier debugging, and faster iteration – beneficial in a field that often requires constant model adjustments and updates.
C++, conversely, has a steeper learning curve due to its complex syntax and the necessity to manage memory manually.
It requires a deeper understanding of computer architecture and programming concepts.
While it offers greater control and efficiency, this comes at the cost of longer development time and potential for errors, especially for those who are less experienced.
What are the advantages of using Python over C++ in quantitative finance?
Python offers several advantages in quantitative finance:
- Rapid Development: Python’s simple syntax allows for quicker development of financial models.
- Extensive Libraries: Python has a rich ecosystem of libraries like pandas, NumPy, and SciPy, which are specifically designed for data analysis and scientific computing.
- Community and Support: Python has a large community, which means abundant resources, tutorials, and forums for troubleshooting and learning.
- Flexibility: Python is well-suited for data exploration, machine learning, and statistical analysis, which are important in quantitative finance.
- Integration Capabilities: Python integrates well with other languages and tools, making it versatile for different aspects of financial analysis.
In what scenarios is C++ preferred over Python for developing financial algorithms?
C++ is preferred in scenarios where performance and speed are critical. These include:
- High-Frequency Trading (HFT): Where microseconds can impact the profitability of trades.
- Large-Scale Numerical Simulations: Where the efficiency of C++ can handle complex calculations more rapidly.
- Systems Closer to Hardware: Such as exchange systems or custom transaction systems where low-level operations are necessary.
- Legacy Systems Integration: In some cases, financial institutions have legacy systems written in C++ that require integration or extension.
How do Python and C++ handle large data sets and real-time data processing in finance?
Python, with libraries like pandas and NumPy, is capable of handling large datasets efficiently.
But its performance can be limited by its interpreted nature, especially in real-time data processing scenarios.
Python’s GIL (Global Interpreter Lock) can also be a limiting factor in multi-threaded applications.
C++, being faster and more efficient at memory management, is better suited for real-time data processing tasks, especially where latency is critical.
Its ability to handle concurrent processes and threads without the overhead of an interpreter makes it more efficient for processing large volumes of data quickly.
Can Python match the execution speed of C++ in high-frequency trading algorithms?
Python typically cannot match the raw execution speed of C++ in high-frequency trading algorithms due to its nature as an interpreted language.
HFT algorithms require extremely low-latency execution, which C++’s compiled nature is better equipped to provide.
However, Python can be used for the development and prototyping phase of these algorithms due to its ease of use before they are translated into C++ for execution.
What are the libraries and tools available in Python and C++ specifically for financial analysis?
In Python:
- pandas: For data manipulation and analysis.
- NumPy: For numerical computing.
- SciPy: For scientific and technical computing.
- scikit-learn: For machine learning.
- Statsmodels: For statistical modeling.
In C++:
- QuantLib: For quantitative finance. (Available in Python too.)
- Boost: Provides a broad range of functionalities including data structures.
- Eigen: For linear algebra, matrix and vector operations.
How does the integration of third-party tools and platforms differ between Python and C++ in finance?
Python easily integrates with numerous third-party tools and platforms, including data sources, web applications, and machine learning platforms.
Its widespread use in various domains makes it versatile.
C++ requires more effort to integrate with third-party tools and platforms.
It might necessitate additional wrappers or interfaces – especially when dealing with high-level applications or web-based services.
What are the security considerations when choosing between Python and C++ for financial software development?
In Python, security concerns mainly arise from its open-source nature.
This could potentially expose code to vulnerabilities.
Python’s large community nonetheless actively works on identifying and patching security issues.
C++ requires careful management of memory and pointers. If mishandled it can lead to vulnerabilities like buffer overflows.
Proper attention to security practices and code audits are important in C++ development.
How do community support and resource availability compare for Python and C++ in the context of financial algorithm development?
Python has a vast and active community, particularly in data science and finance – offering extensive resources, forums, and documentation.
This support network makes Python an attractive option for those developing financial algorithms.
C++, while also having a significant community, may have fewer resources specifically tailored to financial algorithm development compared to Python.
The support is more general to programming and systems development.
What does compiled (how C++ runs) vs. interpreted (how Python runs) mean?
Compiled languages like C++ are translated into machine code before execution, resulting in faster runtimes.
This process involves converting the entire code into a binary executable, which is then run by the computer’s hardware.
Interpreted languages like Python are translated into machine code at the time of execution.
The interpreter reads and executes the code line by line, which generally results in slower execution speeds compared to compiled languages.
This trade-off allows for greater flexibility and ease of debugging in interpreted languages.
Conclusion
The choice between Python and C++ for financial algorithms depends on the specific requirements of the project.
For tasks requiring rapid development, flexibility, and extensive library support, Python is generally the preferred choice.
For scenarios where performance is critical, such as in high-frequency trading, C++ is more suitable.
In many real-world applications, a combination of both is used:
- Python for prototyping and model development, and
- C++ for performance-critical components