Stochastic Programming in Trading & Investing (Coding Example)
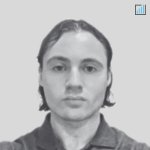
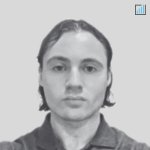
Stochastic programming is a framework for modeling optimization problems that involve unknowns.
In the context of trading and investing, it’s used to make decisions under uncertain market conditions.
The objective is to optimize the expected outcome considering the random variables that represent risks and opportunities in financial markets.
Key Takeaways – Stochastic Programming in Trading & Investing
- Stochastic programming accommodates unknowns in market returns, which helps in decision-making under varied future scenarios.
- It enables the construction of diversified portfolios optimized for expected performance across different market/economic conditions.
- It recognizes that there’s not just a single likely outcome (e.g., stocks return 7% a year), but probability distributions encapsulating many possible outcomes, along with their time evolution and optimizing in light of that.
- By factoring in randomness, it helps traders/investors manage risks and adapt strategies to achieve more stable long-term returns.
- We provide a couple coding examples of stochastic programming later in the article.
Understanding Stochastic Programming
Core Concept
Stochastic programming integrates predictions and uncertainty into mathematical models for decision-making.
It differs from deterministic models by considering multiple scenarios for the future.
This approach acknowledges that there are uncertain outcomes in financial markets and we can’t be sure of future returns, volatilities, etc.
Components
- Decision Variables – Variables that the model will adjust to optimize the objective.
- Objective Function – The goal of the model (usually to maximize returns or minimize risk).
- Constraints – Restrictions or limits on the values that decision variables can take.
- Scenarios – Possible future states of the world, each with a probability of occurrence.
Applications in Trading and Investing
Portfolio Optimization
Stochastic programming helps in:
- Asset Allocation – Determines the optimal mix of assets while considering various economic scenarios.
- Risk Management – Assesses and mitigates risks under different market/economic conditions.
- Liquidity Management – Ensures that the portfolio can meet short-term liabilities without incurring significant losses.
Algorithmic Trading
- Strategy Development – Stochastic models can help in developing trading strategies that adapt to market changes.
- Backtesting – Models are tested against historical data to ensure reliability.
- Execution – If desired, it could be used as part of an algorithmic system to implement trades in an automated and optimized manner. Considers transaction costs and market impact.
Stochastic Programming Techniques
Scenario Analysis
Evaluates a set of possible future events by constructing multiple plausible scenarios.
Each scenario is analyzed to understand its impact on the portfolio or trading strategy.
Stochastic Optimization
Finds the best decision, considering the unknowns in the model parameters.
This can also be extended in some models to hyperparameters and nth-order hyperparameters in probabilities of probabilities scenarios.
It often involves maximizing or minimizing an expected objective, such as expected returns or expected costs.
Monte Carlo Simulation
Uses random sampling to compute results.
It’s widely used in risk assessment and modeling the probability of different outcomes in a process that can’t easily be predicted due to the intervention of random variables.
Math in Stochastic Programming
The basic formulation of stochastic programming is:
- Minimize: cT*x + E[Q(x,ξ)]
- Subject to: A*x ≤ b
Where:
- x = Decision variables that need to be optimized
- c, A, b = Parameters for the linear constraints, as in standard mathematical programming
- Q(x,ξ) = Recourse function that gives the optimal objective value for a given realization ξ of the random parameters
- E[Q(x,ξ)] = Expected value of the recourse function across all probable scenarios
Key Aspects
- There are two types of decisions – here-and-now decisions, x, that are set before uncertainty is resolved, and wait-and-see recourse decisions that can adapt after uncertainty is revealed.
- The randomness or stochasticity is captured through the random parameters ξ and expected value E.
- The wait-and-see decisions are implicitly optimized in the recourse function Q(x,ξ) for a given ξ.
- Determining the expected recourse function for all scenarios is complex, usually requiring Monte Carlo sampling.
So stochastic programming combines optimization, probability and statistics to make the best decisions under uncertainty.
The math brings together a number of fields to tackle this challenge.
Coding Example – Stochastic Programming
We’ll do a stochastic programming optimization on the portfolio we’ve used in our coding examples in other articles:
- Stocks: +5%-7% forward return, 15% annualized volatility using standard deviation
- Bonds: +0%-6% forward return, 10% annualized volatility using standard deviation
- Commodities: +0%-6% forward return, 15% annualized volatility using standard deviation
- Gold: +2%-6% forward return, 15% annualized volatility using standard deviation
There are two main ways to do this in Python – with the cvpxy optimization library and without it (since not all environments support it).
Example #1 – with cvpxy
The Python code defines an optimization problem using stochastic programming principles to determine the optimal allocation of a portfolio consisting of stocks, bonds, commodities, and gold, based on their expected returns and volatilities.
The objective is to maximize the portfolio’s expected return under the constraints that the sum of asset weights equals 1 (fully invested portfolio) and that short selling isn’t allowed (weights are non-negative).
The expected returns of the assets are approximated by taking the average of their given forward return ranges.
This approach simplifies the stochastic nature of the returns into a deterministic optimization problem suitable for cvpxy.
To execute the code in an environment where cvpxy is installed, follow these steps:
- Install cvpxy by running pip install cvpxy.
- Run the provided code snippet. It’ll use cvpxy to define and solve the portfolio optimization problem, and return the optimal weights for each asset class in the portfolio.
This example assumes assets are uncorrelated, simplifying the portfolio variance calculation and focusing solely on optimizing returns under the given constraints.
import numpy as np import cvxpy as cp # Expected return ranges & vol for each asset class expected_returns = np.array([[0.05, 0.07], # Stocks [0.00, 0.06], # Bonds [0.00, 0.06], # Commodities [0.02, 0.06]]) # Gold volatilities = np.array([0.15, # Stocks 0.10, # Bonds 0.15, # Commodities 0.15]) # Gold # Assume returns are stochastic & take the average average_returns = np.mean(expected_returns, axis=1) # Number of assets n_assets = len(average_returns) # Variables for optimization weights = cp.Variable(n_assets) # Objective = Maximize expected portfolio return objective = cp.Maximize(weights @ average_returns) # Constraints constraints = [cp.sum(weights) == 1, # Allocation weights have to add up to 100% weights >= 0] # No short selling # Problem problem = cp.Problem(objective, constraints) # Solve the problem problem.solve() # Find the optimal weights optimal_weights = weights.value optimal_weights
Example #2 (Simplified without cvpxy)
If cvxpy is unavailable, we’ll use a simpler approach to solve the optimization problem without it.
This approach won’t be very sophisticated but can give an idea of how one might approach the problem.
# Define expected returns & volatilities expected_returns = np.array([0.06, 0.03, 0.03, 0.04]) # Average expected returns for each asset volatilities = np.array([0.15, 0.10, 0.15, 0.15]) # Vol for each asset # Number of assets n_assets = len(expected_returns) # Assuming uncorrelated assets simplifies the problem of finding the highest return for a given level of risk. # Here, we'll use a simplistic approach – allocate more to assets with higher expected return-to-volatility ratio. # Calculate return to volatility ratio for each asset return_to_volatility_ratio = expected_returns / volatilities # Allocation based on return to volatility ratio weights = return_to_volatility_ratio / np.sum(return_to_volatility_ratio) weights
And for the sake of showing results, here’s what we get from the code:
- Stocks: 34.29%
- Bonds: 25.71%
- Commodities: 17.14%
- Gold: 22.86%
For more on this type of exercise, we have this article that explores a variety of allocation backtests:
Challenges & Considerations
Computation
Stochastic programming models can be computationally intensive, especially with a large number of scenarios or decision variables.
Advanced computational techniques and software are required.
Model Risk
The accuracy of a stochastic model heavily depends on the assumptions and data used.
Incorrect assumptions or poor-quality data can lead to misleading results.
Overfitting
There’s a risk of overfitting the model to historical data, which can make the model perform poorly in real-time trading or future market conditions.
Stochastic Programming vs. Quadratic Programming vs. Nonlinear Programming vs. Mixed Integer Programming
- Stochastic Programming deals with optimization under uncertainty, incorporating randomness in parameters to model and solve decision-making problems.
- Quadratic Programming focuses on optimizing a quadratic objective function subject to linear constraints. It’s ideal for portfolio optimization and resource allocation problems.
- Nonlinear Programming involves optimizing a nonlinear objective function with or without constraints. Applicable in areas like engineering design and economic forecasting.
- Mixed Integer Programming combines linear programming with integer variables, used for problems requiring discrete decisions, such as scheduling and strategic planning, where solutions must be whole numbers or binary values.
Each method addresses different types of optimization problems for better decision-making across finance.
Conclusion
Stochastic programming provides a structured way to consider uncertainty and complexity.
It’s used for portfolio optimization, risk management, and algorithmic trading.
But the application of stochastic programming requires careful consideration of computational demands, model assumptions, and the risk of overfitting to historical data.