Nonlinear Programming in Trading & Investing (Coding Example)
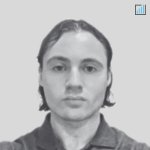
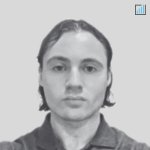
Nonlinear programming (NLP) is a mathematical optimization technique for solving complex problems where the objective function or the constraints are nonlinear.
In trading and investing, NLP is used in portfolio optimization, risk management, and identifying trading strategies that maximize returns or minimize risk.
Key Takeaways – Nonlinear Programming in Trading & Investing
- Better Optimization
- Nonlinear programming allows for more complex, realistic models of market behaviors.
- Enables traders to optimize portfolios with non-linear constraints and objectives.
- Risk Management
- Offers ways for managing portfolio risk by modeling non-linear relationships between assets.
- Adaptive Strategies
- Nonlinear programming supports the development of adaptive trading strategies that can better handle market anomalies and changing conditions.
Portfolio Optimization
Asset Allocation
NLP models help in determining the optimal allocation of assets in a portfolio.
These models consider various constraints and objectives, which makes them suitable for real-world scenarios (rather than purely academic or theoretical exercises).
They can handle nonlinear relationships between assets, and any type of non-linearity, in general.
This accommodates complex risk-return profiles.
Risk Management
Risk management models use NLP to minimize risk for a given level of expected return.
These models factor in the nonlinear behavior of market instruments.
NLP aids in adjusting portfolios in response to market changes, which helps with risk management.
Trading Strategy Development
Strategy Formulation
Traders employ NLP to formulate strategies based on historical data.
By analyzing past performance and market behaviors, NLP models can identify patterns and predict future price movements.
This process involves solving nonlinear equations that represent market dynamics.
Backtesting & Optimization
NLP can be used in backtesting trading strategies to ensure they’re effective before deployment.
It optimizes parameters of a trading strategy by maximizing profitability or other performance metrics.
The nonlinear nature of market data makes NLP appropriate for such optimizations.
Other Factors with Nonlinear Programming
Transaction Costs
NLP can incorporate transaction costs, which are often nonlinear due to commissions, bid-ask spreads, liquidity, and other factors.
This allows for more realistic portfolio optimization and strategy evaluation.
Market Microstructure
NLP can model market microstructure complexities such as market impact, liquidity constraints, and order book dynamics.
This leads to more sophisticated trading strategies that exploit market inefficiencies.
Machine Learning Integration
NLP can be combined with machine learning techniques like neural networks to learn complex relationships from data and develop adaptive trading strategies.
This opens up possibilities for advanced market prediction and anomaly detection.
Alternative Risk Measures
NLP can handle various risk measures beyond standard deviation, such as Value-at-Risk (VaR) and Conditional Value-at-Risk (CVaR), which enables more comprehensive risk management.
Regulatory Compliance
NLP can be used to design portfolios that comply with specific regulatory constraints, such as capital adequacy requirements or sector exposure limits.
Math Behind Nonlinear Programming
The general nonlinear optimization problem is:
- Minimize: f(x)
Subject to:
- g_i(x) ≤ 0, i = 1,…,m (inequality constraints)
h_j(x) = 0, j = 1,…,p (equality constraints)
Where:
- f(x) : R^n → R is the objective function to be minimized over the variable x
- g_i(x) : Inequality constraint functions
- h_j(x) : Equality constraint functions
The problem is to find an optimal point x* that satisfies the constraints and minimizes f(x).
Key aspects
- The functions f(x), g_i(x) and h_j(x) can be nonlinear in terms of x. This distinguishes NLP from linear programming.
- Constraints define the feasible region, and x* will lie on its boundary.
- Methods like Lagrange multiplier theory convert to unconstrained optimization.
- Numerical algorithms like gradient descent, Newton’s method and interior point methods are used to solve NLP problems locally.
- Global optimization through heuristics can attempt to find the global optimum.
In short, the math involves optimizing a nonlinear objective over a nonlinear feasible region described by nonlinear constraints.
Computational algorithms are needed to handle the complex math.
Nonlinear Programming vs. Quadratic Programming
Nonlinear programming can be used for portfolio optimization when the relationship between variables isn’t linear, such as when considering the effects of options or other derivatives, or when incorporating complex constraints.
However, where we have linear returns and constraints (e.g., allocations sum to 100%, no short selling), a quadratic programming approach is more suitable and straightforward.
NLP could be overkill in such cases and unnecessarily complex without a clear non-linear component to optimize.
Challenges & Considerations
Complexity & Computation
NLP problems are often complex and require enough computational resources.
Solving these problems can be time-consuming, and the solutions may be sensitive to initial conditions or parameter settings.
Overfitting
Overfitting is a risk when using NLP.
Models that are too complex/overfitted may perform exceptionally well on historical data but fail to generalize to unseen market conditions.
Practitioners must carefully validate and test their models to ensure robustness.
Market Noise
Financial markets are noisy, and NLP models can be misled by random fluctuations in market data.
It’s important to differentiate between meaningful market trends and noise to avoid making decisions based on spurious patterns.
Data Quality & Availability
The quality and completeness of financial data are important for NLP models.
Missing or inaccurate data can lead to misleading results.
Interpretability & Explainability
Complex NLP models can be difficult to interpret and explain, which can make it challenging to understand the rationale behind their decisions.
Coding Example – Nonlinear Programming
Let’s consider optimizing this portfolio like we’ve used in other articles:
- Stocks: +6% forward return, 15% annualized volatility using standard deviation
- Bonds: +4% forward return, 10% annualized volatility using standard deviation
- Commodities: +3% forward return, 15% annualized volatility using standard deviation
- Gold: +3% forward return, 15% annualized volatility using standard deviation
To illustrate how you might approach this with Python, assuming you want to include a non-linear objective or constraint (for demonstration purposes, let’s assume we aim to minimize risk while also considering some non-linear function of returns), you could use a general-purpose optimizer like scipy.optimize.minimize.
Note that scipy.optimize might not be as efficient for purely quadratic problems as a specialized solver like cvxpy.
Here’s a simple example using scipy.optimize:
from scipy.optimize import minimize import numpy as np # Forward returns & volatilities returns = np.array([0.06, 0.04, 0.03, 0.03]) volatilities = np.array([0.15, 0.10, 0.15, 0.15]) covariance_matrix = np.diag(volatilities ** 2) # Simplified covariance matrix # Objective function: Minimize negative of portfolio return (for demonstration, treating it as non-linear) def objective(x): # Assuming some non-linear relationship for demonstration purposes return -np.sum(returns * x) + np.var(x) # Adding variance to introduce non-linearity # Constraints cons = ({'type': 'eq', 'fun': lambda x: np.sum(x) - 1}) # Sum of weights = 1 bounds = [(0, 1) for _ in range(len(returns))] # No short selling, so weights between 0 & 1 # Random guess, do 1/4 in each x0 = np.array([0.25, 0.25, 0.25, 0.25]) # Perform optimization result = minimize(objective, x0, bounds=bounds, constraints=cons) # Results if result.success: optimized_allocations = result.x print("Optimized Allocations:", optimized_allocations) else: print("Optimization failed:", result.message)
This code snippet sets up a simple optimization problem that could be considered non-linear due to the arbitrary addition of np.var(x) in the objective function.
In real-world scenarios, non-linear elements might involve more complex relationships, including those accounting for, e.g.:
- transaction costs
- taxes, or
- specific financial instruments with non-linear payoff structures
As a key takeaway point:
- The specific non-linear aspect you’d like to optimize should directly influence the design of your objective function and constraints.
Conclusion
Nonlinear programming offers solutions for portfolio optimization, risk management, and strategy development.
However, its effectiveness depends on the careful formulation of problems, rigorous validation of models, and an understanding of market dynamics.