25+ Python Trading Strategies
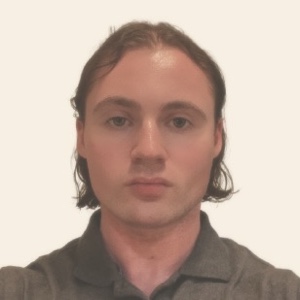
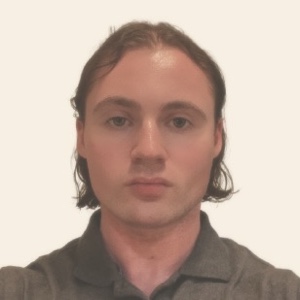
We look at the following Python trading strategies:
- Trend Following
- Momentum Trading
- Swing Trading
- Scalping
- Day Trading
- Position Trading
- High-Frequency Trading (HFT)
- Mean Reversion
- Arbitrage
- Market Making
- Pair Trading
- News Trading
- Algorithmic Trading
- Volume Weighted Average Price (VWAP) Trading
- Dollar-Cost Averaging (DCA)
- Contrarian Investing
- Options Strategies (e.g., Covered Calls, Iron Condor)
- Carry Trade
- Grid Trading
- Martingale System
- Price Action Trading
- Value Investing
- Growth Investing
- Dividend Investing
- Sector Rotation
We’ll explain each strategy briefly and provide a Python sketch for each that codes the basic logic.
For all of these strategies (with the exception of growth investing), we have dedicated pages to each of them that explains in deeper detail.
Python Trading Strategies
1. Trend Following
Trend following involves buying securities that are trending upwards and selling those trending downwards.
It assumes that the trends will continue and is indicative of an underlying, sustainable market shift.
def trend_following(prices): if prices[-1] > prices[-2]: # If the price is trending upwards return "buy" elif prices[-1] < prices[-2]: # If the price is trending downwards return "sell" else: return "hold"
2. Momentum Trading
Traders buy securities that have had high returns over the past three to twelve months and sell those with poor returns.
It’s designed to capitalize on the continuation of existing trends.
def momentum_trading(prices, period=12): momentum = prices[-1] - prices[-period] if momentum > 0: return "buy" else: return "sell"
3. Swing Trading
Swing traders capitalize on swings or fluctuations in asset prices over short to medium terms.
It strives to capture gains in a stock within an overnight to several weeks timeframe.
def swing_trading(prices): # Simple example based on moving averages short_term = np.mean(prices[-5:]) long_term = np.mean(prices[-20:]) if short_term > long_term: return "buy" else: return "sell"
4. Scalping
Scalping involves making very rapid trades to capture small price changes, often entering and exiting trades within minutes.
def scalping(prices, target_profit, stop_loss): buy_price = prices[-1] # Assume price changes current_price = get_current_price() if current_price - buy_price >= target_profit: return "sell" elif buy_price - current_price >= stop_loss: return "sell" else: return "hold"
5. Day Trading
Day trading involves buying and selling securities within the same trading day, not holding any positions overnight.
def day_trading(prices, start_time, end_time): if current_time() < end_time: # Simple strategy based on price change if prices[-1] > prices[-2]: return "buy" else: return "sell" else: return "close all positions"
6. Position Trading
Position trading is a long-term strategy where traders hold positions for weeks to months or even years.
They focus on the asset’s long-term performance.
It’s essentially a hybrid between trading and investing.
def position_trading(prices, long_term_trend): if long_term_trend == "up": return "buy and hold" elif long_term_trend == "down": return "sell or stay out"
7. High-Frequency Trading (HFT)
HFT is a type of algorithmic trading characterized by:
- high speeds
- high turnover rates, and
- high order-to-trade ratios that leverages high-frequency financial data and electronic trading tools
def high_frequency_trading(prices): # Example: arbitrage opportunity detection if prices['Exchange1'] < prices['Exchange2']: return "buy on Exchange1 and sell on Exchange2" else: return "hold"
Related: HFT Strategies
8. Mean Reversion
Mean reversion is based on the assumption that prices will revert back to their historical average or mean.
def mean_reversion(prices, historical_mean): if prices[-1] > historical_mean: return "sell" elif prices[-1] < historical_mean: return "buy" else: return "hold"
9. Arbitrage
Arbitrage involves simultaneously buying and selling an asset or equivalent assets to profit from price discrepancies across different markets or forms.
def arbitrage(price_a, price_b): if price_a < price_b: return "buy A, sell B" elif price_a > price_b: return "buy B, sell A" else: return "no arbitrage opportunity"
10. Market Making
Market makers provide liquidity in the markets by buying and selling securities to facilitate trading.
They earn profits on the bid-ask spread.
def market_making(bid_price, ask_price, target_spread): if ask_price - bid_price > target_spread: return "place buy order at bid, sell order at ask"
11. Pairs Trading (Relative Value)
Pairs trading is a market-neutral strategy that involves taking matching positions in two correlated securities – i.e., buying one and short-selling the other when their price movements diverge.
def pair_trading(asset_a_price, asset_b_price, threshold): # Calculate the price ratio or difference ratio = asset_a_price / asset_b_price if np.abs(ratio - 1) > threshold: if ratio > 1: return "buy B, sell A" else: return "buy A, sell B" else: return "no action"
12. News Trading
News trading involves making trades based on news events, anticipating that the market will move significantly in response to news.
def news_trading(news, current_price): if "positive" in news: return "buy" elif "negative" in news: return "sell" else: return "hold"
13. Algorithmic Trading
Algorithmic trading uses computer algorithms to execute trades at high speeds and volumes based on predefined logic/criteria.
def algorithmic_trading(data, strategy): # Apply a given trading strategy algorithmically return strategy(data)
14. Volume Weighted Average Price (VWAP) Trading
VWAP trading tries to execute orders at a volume-weighted average price to minimize market impact.
Large institutional investors often execute their trades via VWAP when entering or exiting a position, given they may be a significant part of their markets and want to avoid disrupting the market.
Also common when executives with large ownership shares of their company choose to sell.
def vwap_trading(prices, volumes): vwap = np.sum(prices * volumes) / np.sum(volumes) if prices[-1] > vwap: return "sell" elif prices[-1] < vwap: return "buy" else: return "hold"
15. Dollar-Cost Averaging (DCA)
DCA involves regularly investing a fixed amount of money regardless of the asset’s price.
This reduces the impact of volatility.
def dollar_cost_averaging(investment, current_price): # Calculate the number of shares to buy based on fixed investment amount shares = investment / current_price return shares
16. Contrarian Investing/Trading
Contrarian investing is a strategy where investors go against prevailing market trends.
They buy assets when they’re out of favor and sell when they become popular.
def contrarian_investing(market_sentiment, asset_price): if market_sentiment == "very positive": return "sell" elif market_sentiment == "very negative": return "buy" else: return "hold"
17. Options Strategies (e.g., Covered Calls, Iron Condor)
Options strategies involve the use of options contracts to achieve various investment goals, like income generation, generating synthetic leverage, or prudent risk management.
def covered_call(stock_price, strike_price, premium_received): # Example of a simple covered call strategy if stock_price > strike_price: return "exercise option, sell stock" else: return "keep premium"
18. Carry Trade
In a carry trade, an investor borrows money at a low interest rate and invests in an asset that provides a higher return.
They profit from the interest rate differential.
def carry_trade(borrow_rate, investment_rate): if investment_rate > borrow_rate: return "borrow and invest" else: return "do not engage"
19. Grid Trading
Grid trading involves placing buy and sell orders at regular intervals above and below a set price and capture profits as the market fluctuates.
def grid_trading(current_price, grid_size, upper_bound, lower_bound): if current_price > upper_bound: return "sell" elif current_price < lower_bound: return "buy" else: # Adjust grid as needed return "adjust grid"
20. Martingale System
The Martingale system involves doubling down on investments after losses, under the assumption that a winning bet will eventually occur and offset the losses.
def martingale(bet_size, is_previous_bet_lost): if is_previous_bet_lost: return bet_size * 2 else: return bet_size
21. Price Action Trading
Price action trading relies on historical price movements and patterns to make trading decisions without the use of indicators.
def price_action_trading(price_history): # Simple example: buy if price breaks above previous high, sell if below previous low if price_history[-1] > max(price_history[:-1]): return "buy" elif price_history[-1] < min(price_history[:-1]): return "sell" else: return "hold"
22. Value Investing
Value investing involves selecting stocks that appear to be trading for less than their intrinsic or book value.
It emphasizes long-term investment.
def value_investing(stock_price, intrinsic_value): if stock_price < intrinsic_value * 0.8: # Buying at 80% of intrinsic value as an example return "buy" else: return "hold"
23. Growth Investing
Growth investing focuses on companies expected to grow at an above-average rate compared to their industry or the overall market.
def growth_investing(current_growth_rate, expected_growth_rate): if current_growth_rate > expected_growth_rate: return "buy" else: return "hold"
24. Dividend Investing
Dividend investing involves buying stocks of companies that pay high dividends in order to receive regular income.
def dividend_investing(dividend_yield, target_yield): if dividend_yield > target_yield: return "buy" else: return "hold"
25. Sector Rotation
Sector rotation involves moving investments among different sectors of the economy in an attempt to capture the economic and market cycle’s benefits.
def sector_rotation(current_sector_performance, previous_sector_performance): if current_sector_performance > previous_sector_performance: return "rotate to current sector" else: return "stay in previous sector"
Conclusion
These sketches provide a basic framework for each trading strategy.
Actual implementation would include data handling, risk management, performance evaluation, and possibly integration with trading platforms for execution.
The strategies vary widely in complexity, time horizon, risk, and required data.