Deterministic Global Optimization (Trading & Investing Applications)
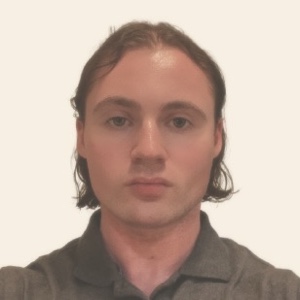
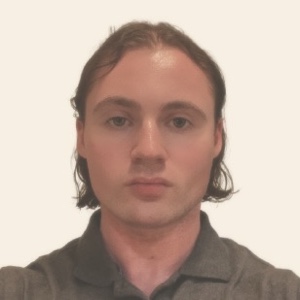
Deterministic global optimization refers to a branch of mathematical optimization that doesn’t rely on probabilistic methods to find the global maximum or minimum of a given function.
In the context of trading and investing, it involves applying rigorous mathematical techniques to optimize trading strategies, portfolio allocations, and trading models with the aim of achieving the best possible performance while managing risk.
Key Takeaways – Deterministic Global Optimization
- Guarantees Global Optima
- Unlike local optimization methods, deterministic global optimization ensures finding the absolute best solution across the entire solution space.
- Useful for maximizing profits or minimizing risks in trading strategies.
- Reduces Uncertainty
- By systematically exploring all possible solutions, it minimizes uncertainty in decision-making.
- Computational Intensity
- The exhaustive nature of the search can require significant computational resources.
- Makes it important to balance precision with practicality in trading applications.
Core Principles of Deterministic Global Optimization
Mathematical Foundations
Deterministic methods use specific conditions or criteria, such as convexity or monotonicity, to systematically search for the optimal solution.
These methods guarantee finding global optima within predefined tolerance levels (given sufficient computational resources).
We look a bit deeper at the math behind deterministic methods in a different section below.
Application in Trading & Investing
In trading and investing, deterministic global optimization is applied to construct efficient portfolios, optimize trading algorithms, and manage risks.
It ensures that the solutions are not just locally optimal (best within a small neighboring set of solutions) but globally optimal (best overall possible solution).
Key Techniques in Deterministic Global Optimization
Branch and Bound Methods
These methods involve dividing the problem into smaller sub-problems (branching) and calculating bounds for the optimal solution in each sub-problem.
If the bound of a sub-problem is worse than the known solution, it is discarded (pruning).
This method is efficient for solving integer and mixed-integer optimization problems, often encountered in portfolio optimization.
Cutting Plane Methods
Cutting plane methods iteratively refine the feasible region of an optimization problem by adding linear constraints (cuts).
These cuts are generated based on the current solution and help in excluding regions that do not contain the optimal solution, thereby improving the search efficiency.
Deterministic Annealing
A technique inspired by statistical mechanics, deterministic annealing progressively refines the solution by considering a series of optimization problems.
Each problem is a softened version of the original, and as the “temperature” parameter decreases, the solution space is gradually restricted to concentrate around the global optimum.
Applications of Deterministic Global Optimization in Trading & Investing
Portfolio Optimization
Deterministic global optimization is a natural fit in portfolio optimization (particularly in the presence of complex, nonlinear constraints and objective functions).
It ensures that the selected portfolio is not just locally optimal but is the best among all possible portfolios, considering the return, risk, and other constraints specified by the trader/investor.
Algorithmic Trading
In algorithmic trading, deterministic optimization techniques are used to develop trading algorithms.
These methods help in fine-tuning the parameters of the trading algorithm to ensure optimal performance across various market conditions.
Risk Management
Deterministic methods are used in risk management by optimizing the allocation of assets to minimize risks such as market risk, credit risk, and operational risk.
They help in constructing portfolios that are resilient to extreme market events and ensure long-term stability.
Math Behind Deterministic Global Optimization
Here’s a bit of background on the math behind deterministic global optimization.
Problem Formulation:
- Minimize f(x)
- Subject to: gi(x) ≤ 0, i = 1,…,m hj(x) = 0, j = 1,…,p LB ≤ x ≤ UB
Where:
- f(x) is the objective function
- gi(x) and hj(x) are inequality and equality constraint functions
- LB and UB are lower and upper bounds on the variables x
Approaches
- Discretization Methods – Sample f(x) at pre-defined discrete points in the domain to find global minimum. Includes grid search, pattern search.
- Interval Analysis – Use interval arithmetic and branch-and-bound techniques to rigorously bracket the global optimum. Allows certification of solution quality.
- Cutting Plane Methods – Iteratively add linear/nonlinear constraints that cut off regions with no optimum. Includes linear programming, Generalized Benders Decomposition.
The math involves formulating theorems/lemmas* to guarantee convergence along with constructive optimization algorithms leveraging special structure to methodically search the entire space.
The key advantage is obtaining provable globally optimal solutions.
The challenges are high complexity for nonconvex problems and the “curse” of dimensionality. Hybridizing with heuristics is often useful to scale.
(*A lemma in mathematics is a proven statement used as a stepping stone toward the proof of a larger result, often serving as a helpful intermediate proposition to build more complex theorems.)
Coding Example – Deterministic Global Optimization
Deterministic global optimization aims to find the global optimum solution to a problem, considering all possible solutions.
In the context of portfolio optimization, one common objective is to maximize the portfolio’s return for a given level of risk, or equivalently, to minimize risk for a given level of return.
Nonetheless, implementing a true deterministic global optimization from scratch can be complex and computationally intensive.
It often requires specialized libraries or algorithms.
For portfolio optimization, a simplified approach can involve discretizing the solution space (i.e., considering a finite set of possible allocations) and evaluating the objective function across this grid to find the best allocation.
Following our common example, let’s say you want to optimize the following portfolio, given this information:
- Stocks: +6% forward return, 15% annualized volatility using standard deviation
- Bonds: +4% forward return, 10% annualized volatility using standard deviation
- Commodities: +3% forward return, 15% annualized volatility using standard deviation
- Gold: +3% forward return, 15% annualized volatility using standard deviation
Below is a Python example that demonstrates a simplified approach to finding an optimal portfolio allocation among stocks, bonds, commodities, and gold (looking to maximize returns for a given level of risk).
This example will use a brute-force search over a discretized allocation space:
import numpy as np # Portfolio assets expected return & standard deviation (volatility) assets_return = np.array([0.06, 0.04, 0.03, 0.03]) # Expected returns assets_volatility = np.array([0.15, 0.10, 0.15, 0.15]) # Vol # "Discretize the solution space" = generate all possible allocations in 10% increments allocation_space = np.array(np.meshgrid(np.arange(0, 1.1, 0.1), np.arange(0, 1.1, 0.1), np.arange(0, 1.1, 0.1), np.arange(0, 1.1, 0.1))).T.reshape(-1, 4) # Filter allocations that sum to 1 valid_allocations = allocation_space[np.sum(allocation_space, axis=1) == 1] # Function to calculate portfolio return & vol def portfolio_performance(weights, returns, volatility): portfolio_return = np.dot(weights, returns) # Simplification: ignoring correlations for volatility calculation portfolio_volatility = np.sqrt(np.dot(weights**2, volatility**2)) return portfolio_return, portfolio_volatility # Target volatility: set a target for portfolio vol target_volatility = 0.10 # Initialize variables to store the best allocation best_return = 0 best_allocation = None # Brute-force search over valid allocations for allocation in valid_allocations: p_return, p_volatility = portfolio_performance(allocation, assets_return, assets_volatility) if p_volatility <= target_volatility and p_return > best_return: best_return = p_return best_allocation = allocation print("Best Allocation:", best_allocation) print("Expected Return:", best_return)
Deterministic Global Optimization vs. Quadratic Programming vs. Nonlinear Programming vs. Mixed Integer Programming vs. Stochastic Programming
Deterministic global optimization focuses on finding the global optimum of an optimization problem with certainty, which ensures that the solution is the absolute best across the entire solution space.
This approach systematically explores all possible solutions.
It makes it particularly effective for problems where local optima aren’t satisfactory and global optima are sought.
DGO is applicable across various types of mathematical functions, including non-linear and complex landscapes, without requiring the problem to be convex.
Quadratic Programming (QP)
QP involves optimizing a quadratic objective function subject to linear constraints.
QP is a specific case of Nonlinear Programming (NLP) where the objective function and constraints are quadratic and linear, respectively.
DGO can solve QP problems but is more general, as QP focuses on problems with a particular form and may not guarantee global optimality unless the problem is convex.
Nonlinear Programming (NLP)
NLP deals with optimizing an objective function subject to equality and inequality constraints, where either the objective function or any of the constraints or both are nonlinear.
NLP techniques often aim to find local optima, and special methods are required to ascertain global optimality.
In contrast, DGO inherently seeks global solutions but can be more computationally intensive than NLP methods tailored for local optimization.
Mixed Integer Programming (MIP)
MIP involves optimization problems where some of the decision variables are constrained to be integers.
An example would be the requirement to buy a whole-integer number of shares of a stock, given an algorithm could tell you to buy a fractional share amount if you don’t put constraints on it.
MIP can handle both linear and nonlinear problems, which makes it versatile for discrete optimization tasks, such as scheduling and allocation problems.
While DGO can address problems with mixed integer variables, MIP specifically targets these discrete aspects and uses specialized algorithms for solving them, such as branch-and-bound or cutting planes, which may not guarantee global optimality in nonlinear cases.
Stochastic Programming (SP)
SP addresses optimization problems involving uncertainty in the data (i.e., stochastic processes).
SP models incorporate randomness within the problem data.
They aim to find solutions that are feasible for multiple scenarios or that optimize the expected value of an objective function.
Unlike DGO, which seeks deterministic solutions, SP explicitly accounts for uncertainty, so it leads to solutions that are robust across different outcomes.
For example, if SP is applied to optimizing a risk parity or “all-weather” approach, it would structure the portfolio such that it could thrive in any market conditions or environment, not just one that’s typically good for equity-centric approaches.
DGO could be applied to deterministic equivalents of stochastic problems or used in a scenario-based approach, but it doesn’t inherently model uncertainty as SP does.
Conclusion
Deterministic global optimization can be used to solve complex optimization problems in trading and investing.
Its mathematical approach ensures that the solutions aren’t just locally optimal but are the best among all possible options.
Related