Fast Fourier Transform Pricing (Applications & Coding Example)
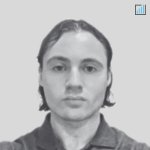
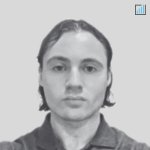
Fast Fourier Transform (FFT) pricing is a computational technique in financial quantitative analysis.
It’s used primarily for the pricing of options and other financial derivatives.
FFT transforms complex pricing models into a more manageable form to perform efficient calculations and analysis.
Key Takeaways – Fast Fourier Transform Pricing
- Computation Efficiency
- Fast Fourier Transform (FFT) speeds up option pricing (notably for a large range of strike prices).
- Flexibility for Complex Models
- FFT is effective in handling complex pricing models that are challenging for traditional methods.
- Example: those with stochastic volatility or jumps
- Accuracy and Precision
- FFT enables precise calculation of option prices, even in sophisticated/non-standard financial scenarios.
- We do a coding example that outlines the basic implementation of the FFT pricing model using synthetic data.
Basics of Fast Fourier Transform
FFT is an algorithm to compute the Discrete Fourier Transform (DFT) and its inverse.
The primary advantage of FFT over DFT is its speed, as FFT significantly reduces the number of calculations needed.
The algorithm transforms time-domain data into frequency-domain data.
In finance, this means converting price movements over time into frequencies or rates of change.
Application in Option Pricing
Challenges with Traditional Methods
Traditional option pricing methods (e.g., Black-Scholes model) can be computationally intensive and less effective for certain types of derivatives.
These models often require numerical methods like Monte Carlo simulations, which can be time-consuming for complex options.
FFT as a Solution
FFT addresses these challenges by simplifying the calculation process.
It allows for the evaluation of option prices under models with complex characteristics, such as jumps or stochastic volatility, more efficiently.
By transforming the problem into the frequency domain, FFT can handle calculations that are otherwise difficult to solve directly in the time domain.
The Process of FFT in Option Pricing
1) Model Specification
The first step involves specifying the option pricing model, which might include factors like stochastic volatility or jump components.
2) Characteristic Function
Instead of directly working with the pricing formula, FFT uses the characteristic function of the option’s payoff.
The characteristic function is a complex exponential that gives the probability distribution of returns (when integrated, as integration sums the area under the curve within certain bounds/constraints).
3) Applying FFT
The FFT algorithm is applied to the characteristic function.
This process converts the complex calculations into a format that is easier to compute.
4) Inverse Transformation
Finally, the inverse FFT is used to transform the frequency-domain data back into the time domain.
This yields option prices.
Advantages of FFT in Pricing
Efficiency
FFT reduces computational time (especially for a large number of strike prices).
Flexibility
It’s well-suited for models where traditional methods are less effective – e.g., those with discontinuous payoff patterns.
Accuracy
FFT can provide accurate results even in complex scenarios.
Limitations & Considerations
Complexity
Understanding and implementing FFT requires a strong grasp of both mathematical and programming concepts.
Model Dependence
The effectiveness of FFT is contingent on the correct specification of the underlying model and its characteristic function.
Numerical Issues
FFT may introduce numerical errors – most commonly if not implemented carefully. So it will require checks for stability and accuracy.
Fast Fourier Transform Pricing
To demonstrate the Fast Fourier Transform (FFT) pricing model in code (in Python, which conveniently has an FFT library within the scipy module), we’ll create a synthetic example (i.e., synthetic data) for option pricing.
The model will be based on characteristic functions and use FFT to compute option prices efficiently.
Let’s consider European call options under the Black-Scholes framework. (American options are more complex due to the early-exercise optionality.)
We’ll create synthetic data for the underlying asset price, strike price, risk-free rate, volatility, and time to maturity.
Steps to Fast Fourier Transform (FFT) in Programming
- Define the Black-Scholes characteristic function – This function is essential for applying FFT.
- Set up parameters – Specify synthetic values for asset price, strike prices, risk-free rate, volatility, and time to maturity.
- Implement FFT – Apply FFT to the characteristic function to compute option prices.
- Inverse FFT – Convert the results back to obtain prices in the time domain.
So let’s set this up:
import numpy as np from scipy.fft import fft, ifft # Black-Scholes Characteristic Function def bs_char_func(u, T, r, sigma): return np.exp(1j * u * (np.log(S0) + (r - 0.5 * sigma**2) * T) - 0.5 * sigma**2 * u**2 * T) # Parameters S0 = 100 # Underlying asset price K = np.linspace(80, 120, num=100) # Range of strike prices T = 1.0 # Time to maturity (1 year) r = 0.05 # Risk-free rate sigma = 0.2 # Volatility # FFT parameters N = 2**10 # Number of points in FFT delta_u = 0.25 # Spacing of FFT grid b = np.log(K[0]) # Lower bound of log-strike # Characteristic function values at different points char_func_values = np.array([bs_char_func(u, T, r, sigma) for u in np.arange(N) * delta_u]) # Apply FFT fft_values = fft(char_func_values) # Adjust & take the real part for option prices option_prices = np.exp(-r * T) * np.real(ifft(fft_values)) / np.pi / 2 # Display option prices for different strikes option_prices_at_K = np.exp(-b) * option_prices / np.exp(np.arange(N) * delta_u * b) # Outputs for i, strike in enumerate(K): print(f"Strike: {strike:.2f}, FFT Option Price: {option_prices_at_K[i]:.2f}")
Note that this is a simplified example for demonstration purposes.
In practice, the implementation might require adjustments for stability, accuracy, and to handle specific features of the financial instruments being priced.
Conclusion
Fast Fourier Transform pricing is useful for the efficient and accurate pricing of complex derivatives.
Its ability to transform difficult time-domain calculations into more manageable frequency-domain computations makes it a valuable technique.
Nonetheless, its application demands an understanding of both the underlying financial models and numerical methods.