Black-Scholes Financial Model in R and MATLAB
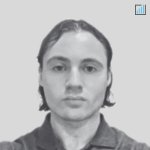
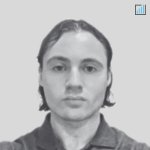
The Black-Scholes model is a mathematical approach toward evaluating the price of an option on an underlying stock/equity. As one of the most accurate option pricing models available, Black-Scholes is still one of the common frameworks by which options prices are modeled and has been in use for over forty years.
It’s derived from an improvement of the earlier Boness model by using the risk-free interest rate as the chosen discount factor and by eliminating assumptions with respect to traders’ and investors’ risk tolerance.
Nevertheless, like any model, there are still assumptions inherent in order to form the foundation of the model.
Black and Scholes made six key assumptions regarding their option pricing model:
1. Efficient Financial Markets
This assumes that markets effectively move in a random manner and that investors cannot accurately determine their direction.
They simply follow a random Markov process in continuous time.
2. The Underlying Asset Pays No Dividends
Dividends throw a wrench into many options models and augment their level of mathematical (and predictive) complexity.
Many companies pay dividends (or a fractional quantity of the stock price) to their shareholders. Consequently, the Black-Scholes model cannot accurately predict options pricing for a dividend-paying underlying.
A higher dividend diminishes the premium on call options in response to the investment having a lesser degree of intrinsic risk for the option seller.
One way to control for the dividend effect in the Black-Scholes pricing model is by taking the current stock price and subtracting the discounted value of a future dividend.
3. Options Cannot Be Exercised Early
American options permit the pre-expiration exercise of an option at any point in its duration. As a consequence, American options have a higher intrinsic value relative to European options, for example, which do not allow early exercise due to the lack of flexibility.
This assumption does not provide a huge discrepancy from the actual, as few options are exercised in the final days before expiry given that the time value of the option doesn’t figure heavily into its price.
4. Transparent and Constant Interest Rates
Black-Scholes uses the “risk-free interest rate,” which is an entirely notional concept as there is inherently no such thing as a “risk-free” investment. All investments carry some degree of risk by nature.
But it represents the theoretical return on an investment under the idea of a genuinely risk-free environment.
The risk-free rate for options pricing is typically taken to be the discount rate on US Treasury Bills somewhere from 1-12 months before expiration. Interest rates regularly fluctuate, which adds some degree of error into the model.
5. No Commisions Charged
In normal scenarios, investors must pay their broker to buy or sell options and they incur transactions costs (difference between bid and ask price).
Trades are exempt from commission fees under the Black-Scholes framework.
6. Returns Will Be Distributed in Lognormal Fashion
Most assets that offer options will have returns that are approximately lognormal, though not precisely so.
This assumption typically does not substantially distort the results of the model.
Mathematical Framework of the Black-Scholes Model
The theoretical prices of a call option, C, and put option, P, can be determined as follows:
C = S * N * (d1) – K * e^(-rt) * N *(d2)
P = K * e^(-rt) – N * (-d2) – S * N * (-d1)
The d1 and d2 sub-variables are defined as:
d1 = [ln(S / K) + (r + sigma^2 / 2) * t] / (sigma * √t)
d2 = d1 – sigma * √t
Where:
- N = cumulative normal distribution function
- S = stock price
- K = option strike price
- r = risk-free interest rate
- t = time until expiration (1 = one year)
- sigma = volatility of stock returns expressed as a standard deviation
- ln = natural logarithm
- e^ = the base of the natural logarithm to the power of (i.e., 2.71828^(…))
The d1 and d2 variables are defined as their own equations and then integrated into the model later for ease of understanding by standard procedure.
The cumulative normal distribution function of d1 denotes a change to the price of the underlying asset.
The cumulative normal distribution function of d2 denotes the risk-adjusted probability that an option will be exercised.
When d1 is multiplied by the stock price, S, this denotes the benefit to buying the underlying in its natural form (i.e., purchasing the stock), as opposed to going with the option.
The Ke^(-rt)*N(d2) provides the present value of paying the exercise price at the point the option expires.
For call options, the appropriate market value is hence a function of benefit to buying the stock outright minus the present value of paying the exercise price when the option expires.
For put options, the call option equation is multiplied by negative one (since puts go in the opposite direction).
Additionally, we change the sign of d1 and d2 to a negative given puts denote the intended direction of an asset going down in price.
In order to create our financial model, we need to define each of our functions above in each program.
That means we will have four equations: one for d1, d2, C (call option price), and P (put option price).
We will define each of our inputs individually in R. We will go through each application individually starting with R.
We will also include the easiest way to code Black-Scholes in MATLAB (i.e., GNU Octave is the free, open-source version), as well.
Black-Scholes Modeling in R
There are a couple of ways to model Black-Scholes in R. Some might opt to use the function() command, but we find the method below to be much simpler and straightforward.
First, we need to define each of our variables for the option we are evaluating, S, K, r, t, and sigma. What we have are mere examples.
We have defined time to maturity at one month (or one-twelfth of a year. For greater precision, this could also be defined based on the specific number of days until expiration (e.g., 30/365).
Finally, we define d1, d2, C, and P.
Writing C on its own in one line of code will provide the value of the object of that name.
In this case, of course, it’s the price of the call option.
The same goes for deriving the price of the put option, P. Writing P on its own line will give you the value/premium of the put option.
S <- 100 K <- 95 r <- 0.05 t <- 1/12 sigma <- 0.25
d1 <- ((log(S/K)+(r+sigma^2/2)*t)/(sigma*sqrt(t))) d2 <- d1-sigma*sqrt(t) C <- S*pnorm(d1,0,1)-K*exp(-1*r*t)*pnorm(d2,0,1) C P <- K*exp(-1*r*t)*pnorm(-d2)-S*pnorm(-d1) P
Black-Scholes Modeling in MATLAB/Octave
The code for MATLAB is similar in structure to what can be used for R.
Here we are defining the Black-Scholes model with the “function” command, including our outputs in parentheses separated by commas, followed by our inputs in parentheses, and separated by commas on the opposite side of the equals sign.
Once d1, d2, C, and P are defined we can “end” our function.
We can write the formulas similar to how we set them up in R.
However, given pnorm() is exclusive to R, we must use the normcdf() function, denoting the cumulative normal distribution function.
function (C, P) = BSM(S, K, r, t, sigma)
d1 = ((log(S/K)+(r+sigma^2/2)*t)/(sigma*sqrt(t)))
d2 = d1-sigma*sqrt(t)
C = S*normcdf(d1)-K*exp(-r*t)*normcdf(d2) P = K*exp(-r*t)*normcdf(-d2)-S*normcdf(-d1)
To call the function in order to obtain our answers, we can type the following into the command window:
[ C, P ] = BSM( 100, 95, 0.05, 1/12, 0.25 )
C =
P =
Note that you can also use alternative names for C and P in the command window. For instance, you could write:
[ Call, Put ] = BSM( 100, 95, 0.05, 1/12, 0.25 )
Call =
Put =
If the order is kept the same relative to how the function was written earlier, the proper outputs will be given even if “C” and “P” are given totally lexically irrelevant names such as Cat and Dog.
Conclusion
The Black-Scholes model is one of the most accurate – and hence one of the most common – financial models for evaluating call and put options on an underlying stock.
There are various software on which this can be modeled.
Excel will be most common in the financial industry, while R and MATLAB will be more common for those doing academic work, statistical analysis, for purposes of creating graphics, or among quants who need to do more sophisticated types of analyses beyond what spreadsheets can typically offer.