Universal Portfolio Algorithm (Principles & Python Example)
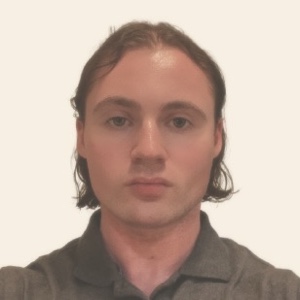
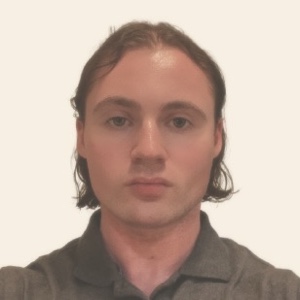
The Universal Portfolio Algorithm is a strategy in quantitative finance developed by information theorist Thomas Cover.
It’s based on the concept of universal schemes in information theory and portfolio selection.
This algorithm aims to achieve long-term growth of capital by effectively distributing investments across a variety of assets in a way that does not rely on predictive models.
Key Takeaways – Universal Portfolio Algorithm
- The Universal Portfolio Algorithm is a portfolio selection strategy that adaptively distributes investment among a set of assets.
- It aims to maximize long-term growth by considering historical data.
- This algorithm operates under the assumption of no prior knowledge about market behavior.
- It leverages mathematical and statistical techniques to continuously update and optimize the portfolio.
- It balances between exploiting known profitable strategies and exploring new investment opportunities (i.e., exploration vs. exploitation).
- We do an example of the Universal Portfolio Algorithm in Python.
Key Principles
Principles of the Universal Portfolio Algorithm:
1. Diversification Over Time
The algorithm continuously updates the portfolio by considering the historical performance of assets.
It adjusts the weight of each asset in the portfolio.
It aims to maximize long-term growth (i.e., log-optimal growth) rather than focusing on short-term gains.
2. Non-parametric Approach
Unlike traditional portfolio management strategies, the Universal Portfolio Algorithm does not make assumptions about the distribution of asset returns or rely on specific statistical models.
It’s a non-parametric approach that uses historical data to guide trading/investment decisions.
Mechanism
Distribution of Wealth
The algorithm begins with a hypothetical set of portfolios.
Each represents a different distribution of wealth across the available assets.
Over time, it observes the performance of these portfolios and gradually shifts the real portfolio towards those distributions that have historically performed well.
Rebalancing Strategy
The portfolio is rebalanced regularly based on the performance of the assets.
This rebalancing is key to capturing the gains from well-performing assets and reducing exposure to underperforming ones.
Significance in Finance
1. Adaptive to Market Conditions
The Universal Portfolio Algorithm is designed to adapt to varying market conditions.
Its reliance on historical performance data allows it to adjust to new market trends and information.
2. Robustness
The strategy is known for its robustness.
Since it does not depend on any specific statistical model or prediction of market behavior (i.e., non-parametric), it avoids the pitfalls of model misspecification or erroneous assumptions about market dynamics.
3. Long-Term Focus
The algorithm is focused on long-term capital growth.
This makes it suitable for investors with a longer investment horizon.
It’s less concerned with day trading and short-term fluctuations, instead concentrating on accumulating wealth over an extended period.
Challenges
Computational Intensity
The algorithm can be computationally intensive, especially with a large number of assets and a long historical data set.
This requires efficient computational methods and resources.
Dependence on Historical Data
While the non-parametric nature is a strength, there’s also a dependence on the quality and quantity of historical data.
Poor or limited data can affect the performance of the algorithm.
Also, the past isn’t indicative of the future in “open systems” like financial markets.
Suitability
The Universal Portfolio Algorithm may not be suitable for all types of investors.
It may not be suitable for:
- day traders or those looking for short-term gains or
- those who have specific risk tolerances that are not aligned with the algorithm’s approach.
Universal Portfolio Algorithm Formula
There isn’t a specific formula for the Universal Portfolio Algorithm.
It’s more about the principles.
We’ll do an example in Python below.
Universal Portfolio Algorithm in Python
Implementing the Universal Portfolio Algorithm in Python requires a combination of data handling, mathematical modeling, and iterative portfolio rebalancing based on historical asset prices.
Below we have a simplified example to demonstrate the concept.
This implementation uses historical stock data and rebalances the portfolio periodically to maximize long-term growth.
Ensure you have the necessary libraries
First, ensure you have the necessary libraries, such as numpy and pandas.
If you don’t have them installed, you can install them using pip:
pip install numpy pandas
Here’s a basic implementation that’s simply an example. Please adjust the code as needed for your own purposes:
import numpy as np import pandas as pd import pandas_datareader as pdr from datetime import datetime # Load historical stock data def load_stock_data(tickers, start_date, end_date): stock_data = pdr.get_data_yahoo(tickers, start=start_date, end=end_date)['Adj Close'] return stock_data # Universal Portfolio Algorithm Implementation def universal_portfolio(stock_data): (n_days, n_stocks) = stock_data.shape portfolio_weights = np.ones(n_stocks) / n_stocks # Equal weights initially portfolio_values = [] for i in range(n_days - 1): # Calculate daily returns daily_returns = stock_data.iloc[i + 1] / stock_data.iloc[i] # Update portfolio portfolio_value = np.dot(portfolio_weights, daily_returns) portfolio_values.append(portfolio_value) # Rebalance portfolio weights portfolio_weights *= daily_returns portfolio_weights /= np.sum(portfolio_weights) return np.array(portfolio_values) # Example usage tickers = ['AAPL', 'MSFT', 'GOOG'] # Example stock tickers start_date = datetime(2005, 1, 1) end_date = datetime(2025, 1, 1) stock_data = load_stock_data(tickers, start_date, end_date) portfolio_values = universal_portfolio(stock_data) # Calculate overall portfolio growth overall_growth = np.prod(portfolio_values) print(f"Overall Portfolio Growth: {overall_growth}")
This code is just a basic framework.
It can be expanded or modified to include more sophisticated rebalancing strategies, risk management techniques, and to handle different asset classes.
The Universal Portfolio Algorithm’s performance will depend on the historical data and the specific rebalancing strategy used.
Related
- Black-Scholes in R and MATLAB
- Best Programming Languages for Algorithm Development
- Best Programming Languages for Portfolio Optimization
FAQs – Universal Portfolio Algorithm
What are universal schemes in information theory and portfolio selection?
Universal schemes in information theory and portfolio selection are strategies or algorithms that perform well across a wide range of scenarios – without the need for specific prior knowledge about data distributions or market conditions.
In portfolio selection, these schemes dynamically adjust asset allocations to optimize returns, based on observed market data.
What is the Universal Portfolio Algorithm and how does it work?
The Universal Portfolio Algorithm is a financial strategy that allocates investments across a basket of assets in a way that adapts to market changes, looking to maximize long-term growth.
It works by analyzing historical price data to continuously adjust the portfolio’s asset distribution.
What are the key advantages of using the Universal Portfolio Algorithm in investment management?
The key advantages of the Universal Portfolio Algorithm include its:
- adaptability to various market conditions
- robust performance without needing prior market knowledge, and
- its ability to learn from historical data to optimize investment decisions
This makes it suitable for a wide range of investment environments.
How does the Universal Portfolio Algorithm handle different market conditions?
The Universal Portfolio Algorithm handles different market conditions by continuously analyzing and learning from historical market data.
It adjusts the investment portfolio in response to observed market trends and fluctuations.
This allows it to adapt to both favorable and adverse market conditions.
What types of assets can be managed using the Universal Portfolio Algorithm?
The Universal Portfolio Algorithm can manage stocks, bonds, commodities, and even complex financial instruments like derivatives.
It depends on the inputs.
Its flexibility allows it to be applied across different asset classes and investment styles.
How does the Universal Portfolio Algorithm differ from traditional portfolio management strategies?
The Universal Portfolio Algorithm differs from traditional portfolio management strategies in its use of algorithmic and statistical methods to dynamically adjust asset allocations based on ongoing market data analysis.
This contrasts with relying on fixed rules or the discretionary judgment of most traders and portfolio managers.
What are the limitations or drawbacks of the Universal Portfolio Algorithm in portfolio management?
The limitations of the Universal Portfolio Algorithm include:
- potential computational complexity
- the need for extensive historical data for accurate analysis, and
- the reliance on past data (the future may not resemble the past)
How is the Universal Portfolio Algorithm different from risk parity?
The Universal Portfolio Algorithm differs from risk parity in that it focuses on maximizing long-term growth by dynamically adjusting asset allocations based on historical price data and trends.
Risk parity aims to allocate assets based on the equalization of risk contributions from each asset class. This often leads to a more static allocation strategy.
While the Universal Portfolio Algorithm adapts to market changes, risk parity primarily balances portfolio risk among different asset types.
Conclusion
The Universal Portfolio Algorithm focuses on long-term growth and robustness against market changes without relying on predictive models.
Its adaptability and non-parametric nature make it an intriguing option for portfolio diversification and management, though it also comes with challenges such as computational demands and data dependency.